Primitive Solids
trCAD offers several ways for generating solid objects. One is the creation of primitive solids that are predefined geometric shapes that can be used as foundation of further constructions. The following primitive types are available:
box | cylinder | cone | torus |
sphere | ellipsoid | wedge |
A detailled description of each of these can be found in the reference section.
Primitive Data Types and Constructors
Each of the different primitives represent a certain data type. This means that a variable of the primitive type can be defined. Primitive type variables need to be initialized by calling a constructor function before they can be used in other functions. If no constructor is called, the variable is uninitialized: it cannot be used directly in other operations but it can be used to receive an initialized value by assignment.
Every primitive type has a default constructor function that takes no arguments. The following example
- creates an uninitialized variable s1 of type sphere
- creates an initialized variable s2 by using the default constructor with nor arguments '()'
- make s1 an initialized variable by assigning it a value from calling a bare default constructor sphere()
Example
sphere s1 // Uninitialized variable s1
sphere s2() // Initialized variable s2
s1 = sphere() // Initialize s1
s1 or s2 could now be used in further operations like the make command.
Beside the default constructor, each primitive type has a number of different constructors that allow the initialization with various settings. As an example, the sphere data type has a constructor that accepts one float as radius and one vector as center position. With this, we can generate spheres of different sizes and positions:
Example
for( int i = 1; i < 10; i++ )
make sphere( i, <[10*i, 0, 0]>)
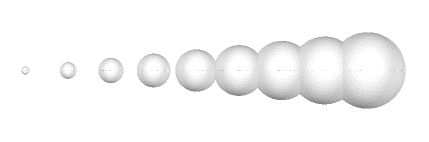
Spheres created by the sphere( float, vector ) constructor with radius and position arguments.
The different constructors are listed in the references of the respective primitives.
The Data Type solid
All primitive data types are a specialization of the general solid data type solid and can be casted into this automatically. That means that the primitive data types can in fact be used in all functions and operators that accept solid types as arguments but a casting to this data type is implied. One example is the make command that works smoothly with a sphere primitive but implicitly casts it to a solid:
Example
sphere s()
make s
Note
The application of modifiers that are explained in a later chapter leads to an implicit convertion into a solid. This means that the variable s in the following example must be defined as solid. Would it be defined as sphere, the compiler would throw an error:
Example
solid s = rgb(64, 244, 124 ) >> sphere()