offset_xy
Creates a sketch by appyling an offset.
Signatures
- offset_xy( curve c, float offset )
- offset_xy( sketch s, float offset )
Details
Constructor (1) projects the curve c to the X-Y plane and applies an offset to to. Constructor (2) applies an offset to sketch s.
Example
curve c = <[0, 0]> -> <[1,0]> -> <[0.5, sqrt(0.75)]> -><-
sketch s = offset_xy( c, 0.5 )
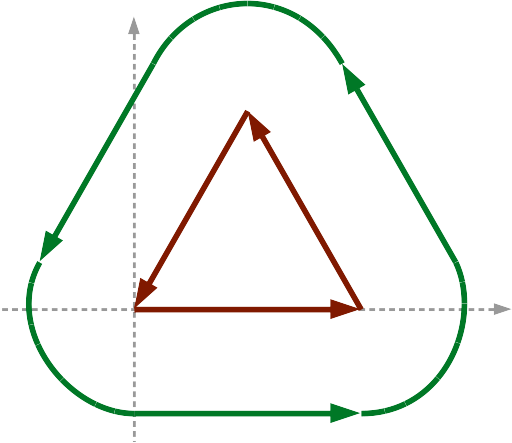
The original curve c (red) from the example and sketch s resulting from an offset of +0.5 (green).
Offsets can be applied to closed and open curves. The following example applies a negative offset to an open curve:
Example
curve c = <[0, 0]> -> <[1,1]> -> <[2, 0]> -> <[2,1]>
sketch s = offset_xy( c, +0.5 )
curve shape = s.element( 0 ) -> -c -><-
make extrusion( shape, <[0,0,1]> )
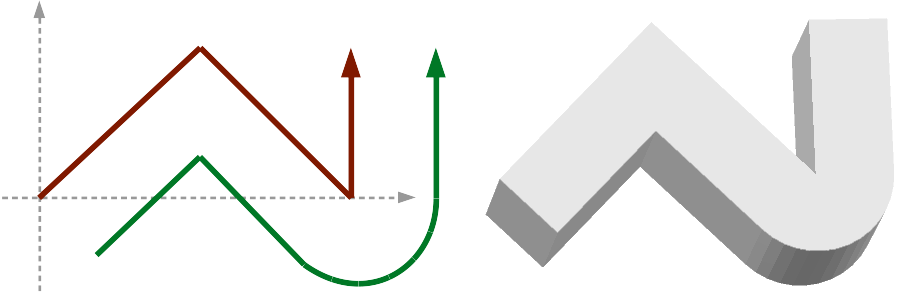
On the left is the curve c (red) and its resulting sketch s (green) from the second example. On the right, the extrusion of shape.
Example
curve c1 = <[8,0]> -> arc( <[0,0]>, 2PI )
curve c2 = <[4,3]> -> arc( <[3,3]>, 2PI )
curve c3 = <[-4,3]> -> arc( <[-3,3]>, 2PI )
curve c4 = <[5,-1.5]> -> arc_to( <[-5,-1.5]>, 5.5, false ) -> arc_to( <[5,-1.5]>, 5.2 )
sketch s = c1 - c2 - c3 - c4
color black = rgb(0,0,0)
color white = rgb(255,255,255)
for( int i = -20; i < 10; ++i )
make ( ( i % 2 == 0 ) ? black : white ) >>
translation( <[0,0,-0.1 * i]> ) >>
extrusion( offset_xy( s, 0.2 * i ), <[0,0,1]> )
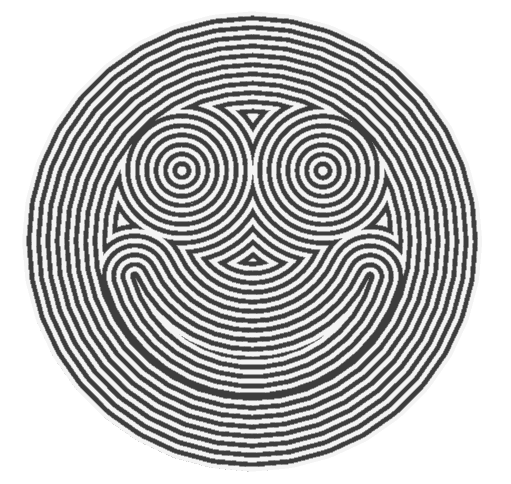
Application of multiple positive and negative of offsets to a smiley sketch.
Return value
A sketch containing the offset of the input geometry.
Parameters
- c
-
A general curve that gets projected onto the X-Y plane before an offset is applied by the function to generate the output sketch.
- s
-
A sketch the offset is applied onto to generate the output.
- offset
-
Offset value that is applied on the input geometry. Positive values are applied into the direction "to the right" of the curves (enlargement of sketched geometries), negative into the direction "to the left" (shrinking of sketched geometries).