Open Parameters
The process commonly described by the term "configuring a 3D model" typically involves the following three steps:
- A user changes one or more parameters of a 3D model.
- The model is updated according to the new parameters.
- The user receives visual feedback.
The trCAD system has a built-in mechanism for defining, setting, and (re-)evaluating user-configurable parameters within a 3D model. In combination with its extension, the trCAD JavaScript API, it can auto-generate a full web-based graphical user interface (GUI) from these parameter definitions.
Such parameters that can be read and set by external users are called open parameters. This section explains their definition and usage. A related concept, public parameters, which can only be read externally, is discussed in the public parameters section.
Definition of Open Parameters
An open parameter is a variable explicitly defined to be readable and writable from the outside. "From the outside" refers to a general external scope that is not visible to the model creator when building the object. Often, this might be an auto-generated user interface, but it could also be another script including the model or a different, unknown system.
The model creator should consider which parameters are best suited for model configuration and how these are designed. The way the parameter influences the model should be easy to understand for someone not involved in the construction. Reducing cross-correlations between different open parameters to a minimum is advisable. Defining open parameters in common units creates a more tangible user experience. The number of open parameters should not be overwhelming for a typical user. These are just a few ideas for parameter design, and there may be many more.
Creating Open Parameters
To create an open parameter, the keyword open needs to be added to its variable declaration. Additionally, an attribute section must follow the declaration. This section contains all mandatory and some optional attributes. In code, this looks like:
Example
open int myopenparameter
{
name = "My Open Parameter"
descr = "This integer value will be usable from within the script"
value = 5
}
echo( myopenparameter )
Output
5
The example defines a variable myopenparameter as an open parameter and sets its three attributes: name, descr, and value. These are mandatory for an integer open parameter. If the trCAD JavaScript API is used, the parameter is automatically created in the control section of the GUI:

An open parameter as it is displayed in the auto-generated web GUI.
The value of the open parameter after its declaration in the example is '5' unless the parameter is changed to another value by the user.
Note
By default, an open parameter is only requested from the user when it is actually used during the runtime of the 3D model script. This means that in the above example, the parameter won't appear in the auto-generated GUI if the last line including the echo is deleted. The idea behind this is to avoid cluttering the control panel with open parameters that have no influence on the 3D model. The visibility rules section explains this concept in more detail.
Open parameters can be generated from certain data types only. The respective references of the data types show whether open parameters can be constructed from them.
Although open parameters can be defined and used in non-global scopes, i.e., inside higher statement blocks, their identifiers must be unique within all open parameters of the package.
Mandatory Attributes
The following three attributes are mandatory for all open parameters:
Attribute | Data Type | Meaning |
name | string | The name of the open parameter that will be visible from the outside. It should describe the parameter briefly. |
descr | string | A more detailed description of the parameter. It should explain the usage and effect of the open parameter. In the default control, this is shown when the user clicks on the "information" icon. |
value | data type of the parameter | The default value of the open parameter that will be used when no other user-specified value is set. |
Note that more mandatory attributes may be required by certain data types, and additional optional attributes may be available. Lists of all additionally available attributes are given in the reference sections of the respective data types.
Interdependent Open Parameters
Simple Dependencies
Some features of an open parameter may depend on the values of other open parameters. This can be modeled by defining the attributes of the open parameter in dependence on the values of other open parameters declared earlier. The following example makes the default value and the optional attribute min of open parameter myopenint2 dependent on open parameter myopenint1:
Example
open int myopenint1
{
name = "My First Open Integer"
descr = "The first integer value."
value = 5
}
open int myopenint2
{
name = "My Second Integer"
descr = "The second integer value. It must be larger than " + myopenint1 + "."
value = myopenint1 + 2
min = myopenint1 + 1
}
echo( myopenint2 )
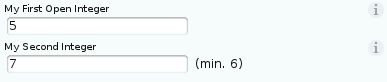
The GUI as it results from the example.
Mutual Interdependencies
Often, two or more open parameters are mutually interdependent. An example would be one value specified in two different units. In this case, the dependency can be explicitly modeled into the script. The changed attribute is used to check whether an open parameter was changed recently. From this, the other open parameters can be adjusted accordingly, as shown in the following example:
Example
open float tempC
{
name = "Temperature [°C]"
descr = "The temperature in degrees Celsius."
value = 0.0
expose_opar = 1
}
open float tempF
{
name = "Temperature [°F]"
descr = "The temperature in degrees Fahrenheit."
value = 32.0
expose_opar = 1
}
if( tempC.changed )
tempF = tempC * 9.0/5.0 + 32.0
if( tempF.changed )
tempC = ( tempF - 32.0 ) * 5.0 / 9.0
echo( tempC + "°C = " + tempF + "°F" )
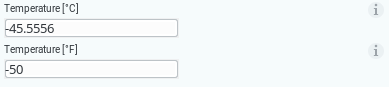
Two mutually dependent open parameters.
Note that in this example it is important to make both open parameters visible since otherwise one parameter would be hidden when it gets set before it is read in the final echo command.
The changed attribute is implicitly available on all open parameters. It has a boolean data type.
Open Arrays
The open keyword can also be used for defining open arrays. The size of the array is left blank in the open parameter declaration since it may vary during use.
Example
int fibonacci[] = [0, 1, 1, 2, 3]
open int sequence[]
{
name = "User sequence"
descr = "Insert a sequence of numbers here"
value = fibonacci
}
echo( sequence )
Using the default value results in the output
Output
<int>[0,1,1,2,3]
Higher Dimension Arrays
Arrays with higher dimensions can be handled in the same way. The number of dimensions must equal the number of [] pairs.
Example
int fibonacci[][] = [[0,1],[1,2],[3,5]]
open int sequence[][]
{
name = "User sequence"
descr = "Insert a sequence of numbers here"
value = fibonacci
}
echo( sequence )
Output
<int>[[0,1],[1,2],[3,5]]