Solids and Primitives
trCAD offers several ways for generating solid objects. One is the creation of primitive solids that are predefined geometric shapes that can be used as foundation of further constructions. The following primitive types are available:
box | cylinder | cone | torus |
sphere | ellipsoid | wedge |
A detailed description of each of these can be found in the reference section.
Primitive Constructors
Each of the different primitives can be created using a constructor function for the general data type solid. This means that a variable of the type solid can be initialized using these constructors. If no constructor is called, the variable is uninitialized: it cannot be used directly in other operations but it can be used to receive an initialized value by assignment.
Every primitive type has a default constructor function that takes no arguments. The following example
- creates an uninitialized variable s1 of type solid
- creates an initialized variable s2 by using the default constructor with no arguments '()'
- makes s1 an initialized variable by assigning it a value from calling a bare default constructor sphere()
Example
solid s1 // Uninitialized variable s1
solid s2 = sphere() // Initialized variable s2
s1 = sphere() // Initialize s1
s1 or s2 could now be used in further operations like the make command.
Beside the default constructor, each primitive type has a number of different constructors that allow the initialization with various settings. As an example, the sphere constructor accepts one float as radius and one vector as center position. With this, we can generate spheres of different sizes and positions:
Example
for( int i = 1; i < 10; i++ )
make sphere( i, <[10*i, 0, 0]>)
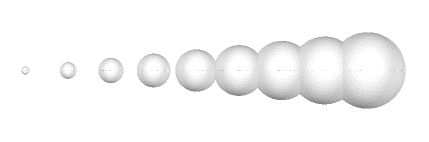
Spheres created by the sphere( float, vector ) constructor with radius and position arguments.
The different constructors are listed in the references of the respective primitives.
The Data Type solid
All primitive constructors return a value of the general solid data type solid. This means that the primitive constructors can be used in all functions and operators that accept solid types as arguments. One example is the make command that works smoothly with a sphere() constructor but implicitly casts it to a solid:
Example
solid s = sphere()
make s